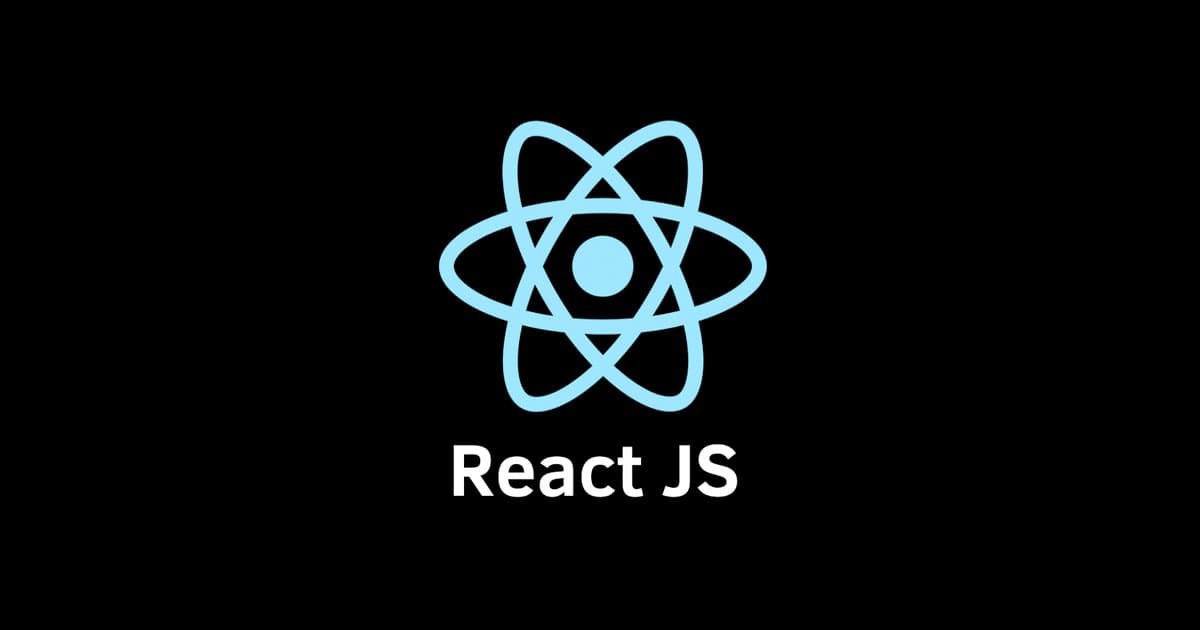
React & JSX Quick Reference Guide
Overview
Concept | Example |
---|
Basic JSX | <div>...</div> |
JSX Component | <Component property={javascript} /> |
Component with Children | <Component>{children}</Component> |
Escaping JavaScript | {...} |
Importing React | const React = require('react'); |
Importing ReactDOM | const ReactDOM = require('react-dom'); |
Rendering in the DOM | ReactDOM.render(<MyApp />, document.getElementById('root')); |
Functional Component | function MyComponent(props) { return <div>...</div>; } |
Base Class for Class Components | React.Component |
Constructor's First Statement | super(props) |
Initializing State | this.state={...} |
Updating State | this.setState({...}) |
Accessing Previous State | this.setState(prevState => ({...})) |
Functional Component Example
'use strict';
const React = require('react');
function Greeting(props) {
return (
<h1>{props.salutation + ' ' + props.who + '!'}</h1>
);
}
const world = 'world';
<Greeting salutation='hello' who={world} />
Class Component with State
class MyComponent extends React.Component {
constructor(props) {
super(props);
this.state = {property: 'initial'};
this.handleClick = this.handleClick.bind(this);
}
render() {
return (
<div>
<button onClick={this.handleClick}>Click me!</button>
<div>{this.state.property}</div>
</div>
);
}
handleClick() {
this.setState({property: 'clicked'});
}
}
Rendering React Component in the DOM
HTML Structure
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>React is awesome!</title>
</head>
<body>
<div id="root"></div>
<script src="https://example.com/transformed-with-babel.js"></script>
</body>
</html>
JavaScript for Rendering
'use strict';
const React = require('react');
const ReactDOM = require('react-dom');
const MyCoolApp = require('./my-cool-app.js');
ReactDOM.render(
<MyCoolApp />,
document.getElementById('root')
);
Component with Children
const borderStyle = {
border: '1px solid darkcyan',
boxShadow: '5px 5px 5px gray',
margin: '10px',
padding: '5px'
};
function Border(props) {
return (
<div style={borderStyle}>
{props.children}
</div>
);
}
Comparison: HTML, Web API, and React JSX
Attribute Type | HTML | Web API | React JSX |
---|
Attribute/Property Names | lower case | mostly camelCase | camelCase |
Attribute Values | string | expression | expression within { } |
Event Handler Names (on...) | lower case | lower case (!) | camelCase |
Event Handler Values | JavaScript string | function expression | function expression within { } |
Preventing Default Behavior | n/a | can return false | must call event.preventDefault(); |
HTML Tags | lower case | n/a | lower case |
Custom Tags (Components) | n/a | n/a | start with capital letter |
Attribute Examples
HTML Attribute | HTML Example | JSX Property | JSX Example |
---|
class | <div class='fancy'>...</div> | className* | <div className='fancy'>...</div> |
onclick | <button onclick="foo()">... | onClick* | <button onClick={foo}>...</button> |
tabindex | <input tabindex=2 /> | tabIndex | <input tabIndex={2} /> |
*Note: className
matches the Web API, whereas onClick
does not! See MDN documentation for further details.
Special Tag Behavior
Tag | HTML Behavior | React Behavior |
---|
<textarea> | content defined by children | content defined by value attribute |
<select> | selection defined by presence of selected attribute | selection defined by value attribute on <select> |
Inline CSS vs. React Inline Style
Aspect | Inline CSS | React Inline Style |
---|
style Attribute/Property | a string enclosed by " or ' | an object enclosed by { } |
Style Property Names | lower case with hyphen - | camelCase |
Style Property Values | CSS expression (textual) | JavaScript expression |
Separator | ; | , |
Merging Styles | no | yes, e.g., with Object.assign() |
Variable Usage | no | yes |
CSS vs. React Style Examples
CSS Property | CSS Inline Style Example | React Style Property | React Inline Style Example |
---|
background-color | style="background-color: green" | backgroundColor | style={{backgroundColor: 'green'}} |
border | style="border: 1px solid black" | border | style={{border: '1px solid black'}} |
border-style, border-width | style='border-style: solid; border-width: 5' | borderStyle, borderWidth | style={{borderStyle: 'solid', borderWidth: 5}} |
Component Lifecycle Methods
Lifecycle Event | Method |
---|
Before Component Unmounts | componentWillUnmount() |
After Component Mounts | componentDidMount() |
Before Component Updates | componentWillUpdate(nextProps, nextState) |
After Component Updates | componentDidUpdate(prevProps, prevState) |
Handy Tips
Tip | Description |
---|
Binding this | Use this.handleEvent.bind(this); in the constructor. |
Unique IDs in Loops | Use <button onClick={(e) => this.method(id, e)}>...</button> or <button onClick={this.method.bind(this, id)}>...</button> |
Conditional Rendering with && | {condition && <SomeComponent>...</SomeComponent>} |
Conditional Rendering with Ternary | {condition ? (<TrueComponent>...) : (<FalseComponent>...)} |
Prevent Rendering | Return null in the render() method. |
Prevent Navigation | Use href="#" or <a href="#" onClick={...}>...</a> |
Reusing Event Handlers | Use a custom name attribute and evaluate it with event.target.name . |
Babel Configuration
Preset: react
Manual Transformation Example
const babel = require('babel-core');
let transformed = babel.transform(code, {"presets": ["react"]}).code;
CSS for Full Page
html, body, #root {
margin: 0;
height: 100%;
width: 100%;
}
This guide should serve as a handy reference for working with React and JSX, maintaining the core code while ensuring a fresh presentation of the material. Sourced and edit from @delibytes Github Git.